One of the first things I had to do, was figuring out how to connect to an external server via Unity client using a TCP/IP socket. It took a lot of searching to find help on this issue but I did find it eventually. I’ve added links to my files here so you can view/use them.
Unity Socket Server A socket server implementation for unity. Can be used with very few lines of code. Basically, can be used to allow clients to connects and send tokens.
So to get your Unity application to connect to an external server via TCP/IP socket, you will need the following:
TCPConnection.cs – the connection handler, server communications
socketScript.cs – the script attached to one of the GameObjects in Unity (I used the Main Camera). We will use this script to attach a copy of TCPConnection.cs to the Camera as well.
I’ve added comments to the important things, hopefully they make sense.
If you want to use javascript instead of C# for your socketScript and application in general, remember to move the TCPConnection.cs into the /Assets/Standard Assets folder (just create it if it doesn’t exist) for it to work. In my case (since I used C#, I just put them both in the /Assets/Scripts folder).
I wrote my own very basic C++ windows socket server to test this. When interacting with server, make sure that your server returns the newline command after every response, otherwise your unity client will be left to hang (until the server disconnects). For our server we had to use n but it depends on what type of server you are running.
- IWebSocket interface for targeting the various platforms Unity supports. WebSocketMono utilizes WebSocket-Sharp. and should work on all mono platforms including the Unity Editor on Mac and PC. WebSocketUWP utilizes MessageWebSocket for Windows 10 (UWP) apps.
- Important: UNet is a deprecated solution, and a new Multiplayer and Networking The Unity system that enables multiplayer gaming across a computer network.
If you want to have multiple connections (like for most games, you’ll have the login server and the game/world server), you just got to add another instance of TCPConnection.cs to your socketScript.

Moving notice:
I wasn’t gonna bring over both posts separately, so this already includes the updates for multiple messages at once and using the split method to separate them. Also I’m now hosting these files here on this actual server instead of my synamicdsolutions URL.
Example
Unity networking provides the High Level API (HLA) to handle network communications abstracting from low level implementations.

In this example we will see how to create a Server that can communicate with one or multiple clients.
The HLA allows us to easily serialize a class and send objects of this class over the network.
The Class we are using to serialize
This class have to inherance from MessageBase, in this example we will just send a string inside this class.
Creating a Server
We create a server that listen to the port 9999, allows a maximum of 10 connections, and read objects from the network of our custom class.
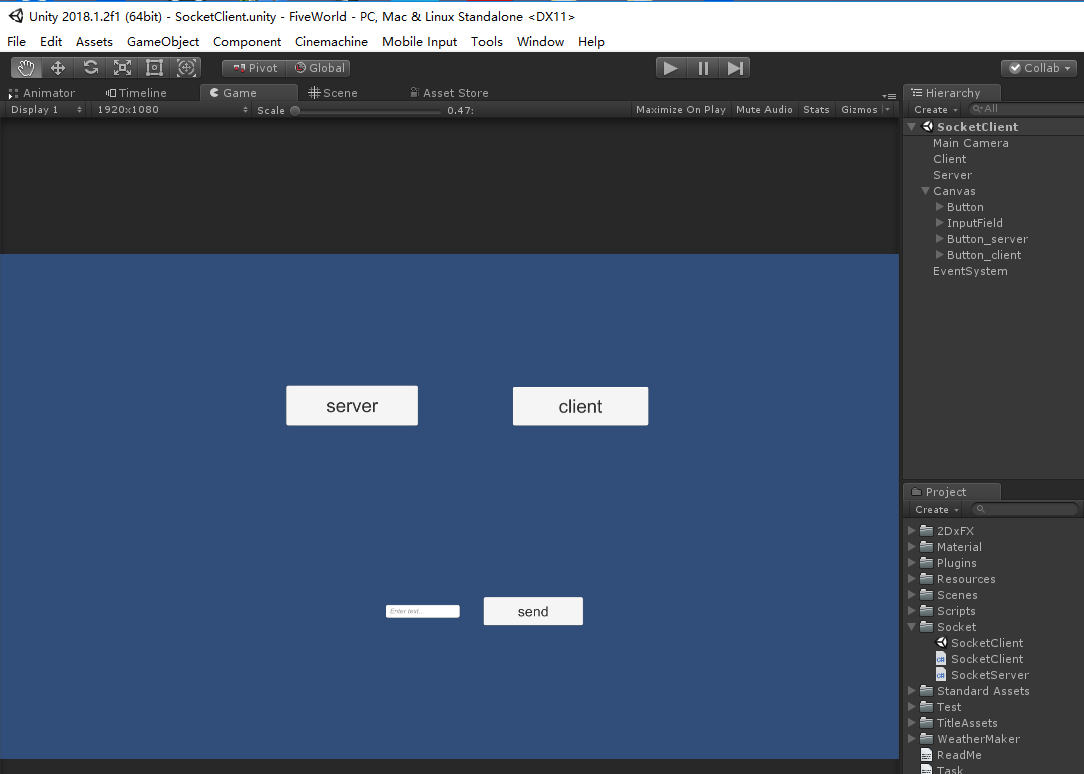
The HLA associates different types of message to an id. There are default messages type defined in the MsgType class from Unity Networking. For example the connect type have id 32 and it is called in the server when a client connects to it, or in the client when it connects to a server. You can register handlers to manage the different types of message.
When you are sending a custom class, like our case, we define a handlers with a new id associated to the class we are sending over the network.
Unity 3d Networking
The Client
Unity Tcp Socket
Now we create a Client
Unity Socket
Unity Socket Sets
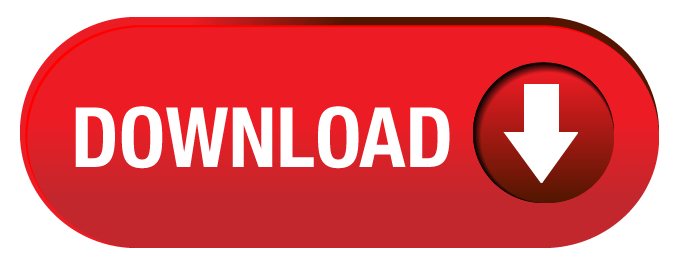